org.perfectjpattern.core.api.structural.composite
Interface IComposite<C>
- Type Parameters:
C
- Component element type
- All Superinterfaces:
- Collection<C>, InvocationHandler, ISurrogate<C>, Iterable<C>, List<C>
- All Known Implementing Classes:
- Composite
public interface IComposite<C>
- extends ISurrogate<C>, List<C>
Composite Design Pattern: Compose objects into tree structures to
represent part-whole hierarchies. Composite lets clients treat individual
objects and compositions of objects uniformly. (Gamma et al, Design Patterns)
Responsibility Abstract definition of the "Composite":
- defines behavior for components having children.
- stores child components.
- implements child-related operations in the Component interface.
Example usage:
//
// Define a Component type interface (or use an existing one).
//
interface IComponent
{
//--------------------------------------------------------------------
public void
printValue(String aValue);
}
//
// Implement a simple component.
//
class Component
implements IComponent
{
//--------------------------------------------------------------------
public void
printValue(String aValue)
{
System.out.println(aValue);
}
}
// create four Component instances
IComponent myComponent1 = new Component();
IComponent myComponent2 = new Component();
IComponent myComponent3 = new Component();
IComponent myComponent4 = new Component();
// create Composite instance 1
IComposite<IComponent> myComposite1 =
new Composite<IComponent>(IComponent.class);
// create Composite instance 2
IComposite<IComponent> myComposite2 =
new Composite<IComponent>(IComponent.class);
// build compositions
myComposite1.addAll(myComponent1, myComponent2);
// also nest compositions
myComposite2.addAll(myComponent3, myComponent4, myComposite1.
getComponent());
// call method on the Composite Component view that triggers
// execution over the complete composition
myComposite2.getComponent().printValue("Hello World!");
- Version:
- $Revision: 1.0 $ $Date: Nov 18, 2007 9:51:14 PM $
- Author:
- Giovanni Azua
-
-
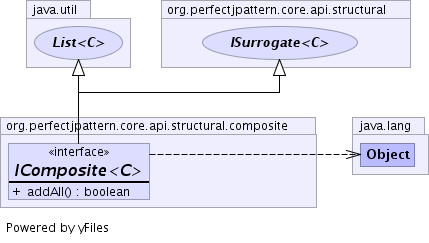
Method Summary |
boolean |
addAll(C... anElements)
Appends all of the elements in the specified array to the end of
this list, in the order that they are returned by the specified
collection's iterator (optional operation). |
Methods inherited from interface java.util.List |
add, add, addAll, addAll, clear, contains, containsAll, equals, get, hashCode, indexOf, isEmpty, iterator, lastIndexOf, listIterator, listIterator, remove, remove, removeAll, retainAll, set, size, subList, toArray, toArray |
addAll
boolean addAll(C... anElements)
throws IllegalArgumentException
- Appends all of the elements in the specified array to the end of
this list, in the order that they are returned by the specified
collection's iterator (optional operation). The behavior of this
operation is undefined if the specified collection is modified while
the operation is in progress. (Note that this will occur if the
specified collection is this list, and it's nonempty.)
- Parameters:
anElements
- Array containing elements to be added to this list
- Returns:
- true if this list changed as a result of the call
- Throws:
UnsupportedOperationException
- if the addAll operation
is not supported by this list
IllegalArgumentException
- if the specified collection contains one
or more null elements, or if the specified collection is null
IllegalArgumentException
- if some property of an element of the
specified collection prevents it from being added to this list
IllegalArgumentException
- 'anElements' must not be null
IllegalArgumentException
- 'anElements' must not be empty
IllegalArgumentException
- 'anElements' must not contain null
Elements- See Also:
List.add(Object)
Copyright © 2007-2009. All Rights Reserved.