org.perfectjpattern.core.api.behavioral.observer
Interface IObserver<E>
- Type Parameters:
E
- Type of event data that this IObserver
subscribes
to.
- All Known Subinterfaces:
- IClock
- All Known Implementing Classes:
- AnalogClock, DigitalClock, ObserverProxy
public interface IObserver<E>
Observer Design Pattern: Define a one-to-many dependency between
objects so that when one object changes state, all its dependents are
notified and updated automatically. (Gamma et al, Design Patterns)
Responsibility: Abstract generic definition of the "Observer".
pattern role.
- Defines an updating interface for objects that should be notified of
changes in a subject.
Notes:
Improves over the original GoF recipe and Java Observer implementation
in that:
- Push over Pull approach:
IObserver
instances are not
coupled to the ISubject
interface i.e. there is no need for the
IObserver
to query ISubject
's state. State
change or Message content is pushed to the update(E)
callback
method.
- Strongly typed generic parameter eliminates the need for
IObserver
to downcast the parameter as is the case for
e.g. java.util.Observer
implementation.
Example usage:
//
// Create Observer Pattern elements
//
ISubject mySubject = new ConcreteSubject();
IObserver myFirstObserver = new ConcreteObserver();
IObserver mySecondObserver = new ConcreteObserver();
//
// Subscribe multiple Observer instances to the Subject
//
mySubject.attach(myFirstObserver, mySecondObserver);
//
// Notify all subscribed Observers, optionally pass some Event
// data associated with the Event.
//
mySubject.notifyObservers(NullEventData.getInstance());
//
// Detach Observer instances
//
mySubject.detach(myFirstObserver);
- Version:
- $Revision: 1.0 $ $Date: Jun 11, 2007 6:16:01 AM $
- Author:
- Giovanni Azua
-
-
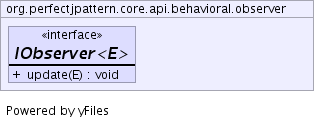
Method Summary |
void |
update(E anEventData)
Notification from ISubject instance that a change
has ocurred. |
update
void update(E anEventData)
- Notification from
ISubject
instance that a change
has ocurred.
- Parameters:
anEventData
- Event data pushed to IObserver
instances.
Copyright © 2007-2009. All Rights Reserved.